In JavaScript, to compare two arrays we need to check that the length of both arrays is the same, the objects present in it are of the same type and each item in one array is equal to the counterpart in a different array.
In this article, we shed light on “How to compare arrays in JavaScript”.
Arrays may need to be compared for determining equivalency. Arrays in JavaScript are different than arrays in C or C++.
Arrays can have a number or a text index in JavaScript. Using the numbered index, traversing, and comparing arrays is easy but it’s complicated in text indexes.
Let’s see a few of the possible ways to compare arrays in different scenarios:
- The arrays have a numbered index and are equal in length.
- The arrays have a numbered index and are not equal in length.
- The arrays have a text index and are equal in length.
- The arrays have a text index and not equal in length.
The Arrays have a Number index and are Equal in Length
For instance, we have two arrays as below, and we want to compare these arrays.
var Array1 = {"1", "2", "3"} var Array2 = {"1", "2", "4"}
The above arrays have a small number of elements, which is three (3). Determining equivalency for the small array is easier.
But for longer arrays, we have to find some method to find it. JavaScript comes with a built-in length method that can be used with arrays and objects to find their length.
This length can be compared using a conditional construct preferably an if statement. This would be something like this:
if(Array1.length == Array2.length){ // do something; }
Comparing the length is necessary as we are dealing with the case of Arrays with equal size. If arrays length is equal then cross elements of arrays could be easily checked using a loop preferably a for–loop.
This would be something like this:
for.... { document.write("Array 1 element " + Array1[i] + "is equal to "+ "Array 2 element " + Array2[i]); }
This will just output some text statements comparing the cross elements. This could be modified to output some text like “Arrays are equal” to make it simple.
There could also be an else part that can handle the case of arrays not being equal.
The Arrays have a Numbered Index and are not Equal in Length
As we have mentioned in the else part of the previous scenario, Arrays may not be equal in length. In such a case, Array 1 may be equal to Array 2 or but Array 2 may not be.
For example, Array 1 has three elements as we have previously used but Array 2 has 4 elements. Array 2 have all of the element of Array 1 but that`s not true of the Array 1.
For such a case we may compare each element of an Array with each element of the other array and another way around.
But in this case, only one array can be equal to the other or not but both can’t be equal as they have varying lengths. For comparing the arrays in this fashion, we can use nested for loop which could be something like this:
for... for... { { if(Array[i] == Array[j]) { //do something } } }
We must use an if statement within the nested loops as we are dealing with arrays of varied sizes.
The Arrays have a Text Index and are Equal in Length
Text indexed arrays is not a good practice. It further complexes the task of even comparing arrays of length as well as unequal length. This also covers “The arrays have a text index and not equal”.
The same Length, Each Value is Equal
One approach for comparing var1 and var2 is checking if each value of var1 is strictly equal to the corresponding value of var2.
This works well if all the elements of the arrays are primitives as opposed to objects. End of the operation it returns a boolean value. Here is an example:
const var1 = [1, 2, 3]; const var2 = [4, 5, 6]; const var3 = [1, 2, 3]; function arrayEquals(var1, var2) { return Array.isArray(var1) && Array.isArray(var2) && var1.length === var2.length && var1.every((val, index) => val === var2[index]); } arrayEquals(var1, var2); arrayEquals(var1, var3);
The output should look like this:
false
true
Using the JSON.stringify() method
JavaScript provides a function JSON.stringify() in order to convert an object or array into JSON string. By converting into JSON string we can directly check if the strings are equal or not.
However, the JSON.stringify() method converts an array into a JSON string. Have a look at the below program to understand the concept well.
function compareArrays(arr1, arr2){ // compare arrays const result = JSON.stringify(arr1) == JSON.stringify(arr2) // if result is true if(result){ console.log('The arrays have the same elements.'); } else{ console.log('The arrays have different elements.'); } } const array1 = [1, 3, 5, 8]; const array2 = [1, 3, 5, 8]; compareArrays(array1, array2);
The above program will compare the given arrays and produce an output. The arrays have the same elements because we put the same value in both of the arrays.
In this blog post, we have learned “How to compare arrays in JavaScript” and looked into some of the available methods to do so including straightforward example code.
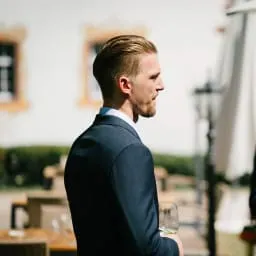
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!