How can you restart your Python program from within itself? Well, it is quite simple. You only need to add one line to your program.
Let’s do this using an example. Suppose we have a program that takes a score from the user and tells the remarks.
For example, if the score is 90, then the remark would be outstanding. If the user enters the score correctly, then the program will run properly.
Moreover, for a score to be correct, it must be a number and in the range of 0-100.
Now, if the user enters an invalid score, we want the program to display the error message and then restart again. We can easily do so using the following line of code.
subprocess.call([sys.executable, os.path.realpath(__file__)] + sys.argv[1:])
Make sure to import sys, os, and subprocess before using the above line.
The complete code is given below.
import os import sys import subprocess def calculateGrade(): try: val = float(input("Enter your marks: ")) if val >= 90 and val <= 100: print("Outstanding") elif val >= 80 and val < 90: print("Excellent") elif val >= 70 and val < 80: print("Very Good") elif val>= 60 and val < 70: print("Needs Improvement") elif val>=30 and val <60: print("Work hard") elif val>=0 and val<30: print("Poor") else: raise ValueError("Enter a valid score, i.e., between 0 and 100") except Exception as err: print("ERROR:", err) print("Restarting the program") print("------------------------") subprocess.call([sys.executable, os.path.realpath(__file__)] + sys.argv[1:]) #restart the program calculateGrade()
Output
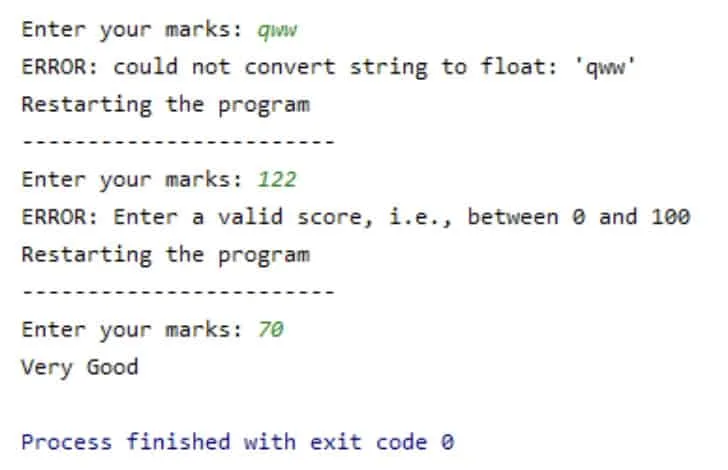
Python Restart a program output
In the above example, when the user enters an incorrect input, an exception gets raised. It gets handled in the except block, where we display the error message and restart the program.
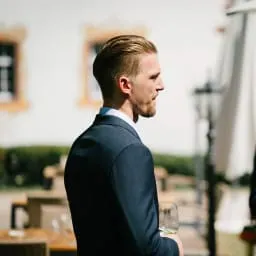
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!