Today, we will see how to check if a letter is uppercase in Python. You can easily do this using Python’s isupper() method.
The syntax is str.isupper(), i.e., it is invoked on the string that we want to check. It takes no parameters, and it returns True if all the characters in a string are capitalized.
If even one of the letters is lowercase, then it will return False. For this problem, we only have a single character.
Therefore, it will return True if the letter is uppercase. Otherwise, it will return False.
Let’s have a look at an example.
letter = "A" is_upper = letter.isupper() if is_upper: print(f"{letter} is in uppercase") else: print(f"{letter} is not in uppercase")
Output
A is in uppercase
Consider another example.
letter = "o" is_upper = letter.isupper() if is_upper: print(f"{letter} is in uppercase") else: print(f"{letter} is not in uppercase")
Output
o is not in uppercase
If we invoke this method on an empty string (no character) or a string that does not have any English alphabets, then it will return False. Let’s see.
checks=["", " ", "@", "MY NAME IS @", "2@!"] for string in checks: is_upper = string.isupper() if is_upper: print(f"'{string}' is in uppercase") else: print(f"'{string}' is not in uppercase")
'' is not in uppercase ' ' is not in uppercase '@' is not in uppercase 'MY NAME IS @' is in uppercase '2@!' is not in uppercase
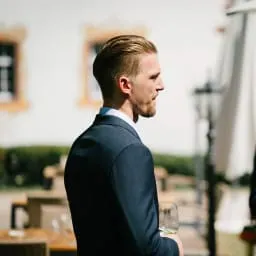
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!