Python strings are immutable. Once you create a string, you can not change or modify the string in Python programming.
Python strings become a not compromisable datatype while working in a real-time Python project. It is often used in any kind of development, and it doesn’t matter if it is a desktop or web-based application!
Our quest is to find ways to check if a string is empty in Python. This article also sheds light on the problem of strings operation and its solution.
At the end of this article, you will know how a string can be checked for being empty or full of whitespaces using different Python methods.
Please note: a string with spaces is actually an empty string but has a non-zero size in Python.
Fortunately, we have several methods and ways available in Python to check a string’s status.
Here are the possible approaches to check if a string is empty in Python.
How to Check if a String is Empty in Python
- Using the len() method
- Using the not
- Using the not + str.strip() method
- Using the not + str.isspace method
- Using the __eq__ method
Using the len() method
The len() method is the most naive and generic method to verify a string’s emptiness to achieve our goal.
The len() is a simple method that checks zero-length string. This method ignores that a string with just spaces should also be practically considered an empty string, even if it is non-zero.
Here is an example of the len() method to check a Python string.
my_str1 = " " my_str2 = " " print ("Is the zero-length string without spaces empty? : ", end = "") if(len(my_str1) == 0): print ("Yes") else : print ("No") print ("Is the zero-length string with just spaces empty? : ", end = "") if(len(my_str2) == 0): print ("Yes") else : print ("No")
The output should look like the following:
Is the zero-length string without spaces empty? : Yes
Is the zero-length string with just spaces empty? : No
Using the not method
The not operator is another helpful operator to check a string’s emptiness. Like the len() method, it checks for the zero-length string and returns the value under an if-else statement.
An example is given below for a better understanding.
my_str1 = "" my_str2 = " " print ("Is the zero-length string without spaces empty? : ", end = "") if(not my_str1): print ("Yes") else : print ("No") print ("Is the zero-length string with just spaces empty? :", end = "") if(not my_str2): print ("Yes") else : print ("No")
Output:
Is the zero-length string without spaces empty? : Yes
Is the zero-length string with just spaces empty? : No
Using the not + str.strip() method
The strip() method is a helpful method, which returns a boolean value. The problem of empty + zero-length string can be resolved by applying the strip() method.
This method returns true when it encounters the space in a zero-length string. Follow the below snippet to get it clearly.
my_str1 = "" my_str2 = " " print ("Is the zero-length string without spaces empty? : ", end = "") if(not (my_str1 and my_str1.strip())): print ("Yes") else : print ("No") print ("Is the zero-length string with just spaces empty? : ", end = "") if(not(my_str1 and my_str2.strip())): print ("Yes") else : print ("No")
Output:
Is the zero-length string without spaces empty? : Yes
Is the zero-length string with just spaces empty? : Yes
Using the not + str.isspace method
This method works almost the same as the previous one. In terms of efficiency, this method is more efficient.
This method doesn’t need to perform the strip() check. Let’s implement this method in a Python program to see how it works.
my_str1 = "" my_str2 = " " print ("Is the zero-length string without spaces empty? : ", end = "") if(not (my_str1 and not my_str1.isspace())): print ("Yes") else : print ("No") print ("Is the zero-length string with just spaces is empty? : ", end = "") if(not (my_str2 and not my_str2.isspace())): print ("Yes") else : print ("No")
Output:
Is the zero-length string without spaces empty? : Yes
Is the zero-length string with just spaces is empty? : Yes
Using the __eq__ method
The __eq__ dunder method is a comparison-based function. It compares two objects of a class. It can also be used for a customized comparison.
In our case, we will check our two different strings through this method. Let’s see how it works.
my_str1 = "Peter" my_str2 = "" if "".__eq__(my_str1): print("It is an empty string") else: print("It is a non-empty string") if "".__eq__(my_str2): print("It is an empty string") else: print("It is a non-empty string")
Output:
It is a non-empty string
It is an empty string
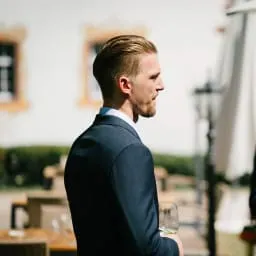
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!