Today, we will shed light on “How to square a number in JavaScript.” Please note that the number may be provided as a constant assigned to a variable or can be provided dynamically using a form.
We will be writing a general JavaScript program to square any number irrespective of it being positive or negative. Let’s start.
The very first step would be writing the basic syntax of a JavaScript program. JavaScript usually resides inside the head tag of an HTML document.
JavaScript Syntax
Therefore, we will use it inside of the head tag instead of the body. JavaScript starts with a <script> tag and ends with </script> tag. The basic syntax is given below.
<html> <head> <script> ........................... </script> </head> <body> </body> </html>
Create a Function to Square a Number in JavaScript
We can write a square performing code directly inside the script tag, but it is better to create a function and write code inside to be reused.
A JavaScript function is declared with a function succeeded by the function name. The general syntax of the JS function looks like below:
function functionName(parameter1, parameter2) { function body; }
If we write out the Square function, it will start with the keyword “function,” and its name can be any, but it is better to use self-explanatory names, so we will use the name “Square.”
function Square(parameter1, parameter2) { function body; }
Inside the basic syntax, we have given two parameters, and these can be any number depending on the need.
Parameters are the values passed to the function to work on them and return the result after the calculation.
However, we aim to create a function that accepts one parameter and returns the given number’s squared value.
If we try to understand it from our real-life example, it’ll be more straightforward. We want to calculate the number’s square.
What needs to be provided for the calculation? Nothing but a number. A function can also be thought of as a machine that takes something, processes it, and outputs something.
In this case, the function will take a number as input, process it, and output the Squared number. So, after writing the parameter for our function, the function would be something like this:
function Square(number) { function body; }
Now, the keyword function tells JavaScript that this part of the code is a function. The word Square is the function name we have chosen for this function, and this can be anything but better self-explanatory.
The parenthesis part denoted by ( ) encloses the value we give to the process function, and we also name it. This is called a variable as it can have different values.
Here we have named variable as a number as this is self-explanatory; otherwise, it could have anything. After that, we have braces denoted by { }; these just enclose the function’s body.
The body of the e function is only the code that does processing on the given value. So far, we have discussed all parts of the JS function, and now we will be going to learn the body of the function in detail.
When we get a number for squaring, what are we supposed to do or imagine when we give a number to a machine-like calculator?
What is it supposed to be done? A calculator takes the input from a user and returns the value immediately after pressing the equal-signed button.
Here, we are going to do the same thing using the JavaScript language. Let’s observe what we have when the JavaScript function started. At the beginning of the function, when the compiler reads the code.
We already know that the function keyword will just tell that this piece of code is a function succeeded by the name of the function and then the number in parenthesis to be worked on, and finally, the body of code in braces.
What the Compiler does
When the compiler starts reading the code, it gets the first curly brace, and then it finds a number stored inside the variable number to work on.
How to Square a Number
Let’s overlook programming for a while and think about how we can square a number in general.
When a number is multiplied by the number itself, a square value is produced.
Therefore, we can define it simply by saying square of a number = number multiply by the same number.
How to Multiply in JavaScript
Hence, JavaScript represents multiplication with the symbol * so that the above statement would be as the square of number = number * number.
Here, the = sign is called an assignment operator and is assigned the value on the right to the left, whether it be a single constant value, a variable, or an expression.
The expression would be evaluated first and then assigned to the variable to the left. I hope now we understand the basic concept of squaring a number and can implement it into JavaScript. The code will be something like this:
function Square(number) { var Square; Square = number * number; return Square; }
How To Simplify the JavaScript Syntax
Let simplify the above snippet. What we have done from the line var Square; we have actually declared a variable for storing the square of the desired number.
Then we have assigned the square to it by using the statement square = number * number; please note that every JavaScript statement ends with a semicolon.
A question could be raised at the last line. What is the purpose of using return Square;? We are returning the value stored in the Square variable from the function.
It is like we give something to the machine, and machines give something back in a different form. Like we provide grains for a grinding machine, and it provides us with a flour.
Here grains are the variable number we give to the function, and it will return the value stored in the variable square, which is the square of the given number. Let’s see the complete program inside HTML code.
<html> <head> <script> function Square(number) { var Square; Square = number * number; return Square; } document.getElementById("square").innerHTML = Square(4); </script> </head> <body> <p id ="square"></p> </body> </html>
document.getElementById(“square”).innerHTML = Square(4); , this line is actually referring to the HTML element with Id “square” and changing its contents to the result of the function.
In this state, we still don’t get the changes on our browser because we have declared function in the head, but we are trying to change the content of HTML tag <p id=”square”></p> in the body.
The browser reads the code from left to right and top to bottom so when it reads the line document.getElementById(“square”).innerHTML = Square(4); it doesn’t know of the tag with the id “square”.
A simple solution is just moving the JavaScript part to the end of the code after </html>. Let’s do it.
To square a number in Javascript, use this code:
<!DOCTYPE html> <html> <head> </head> <body> <p id="demo"></p> </body> </html> <script> function square(number) { var square; square = number * number; return square; } document.getElementById("demo").innerHTML = square(5); </script>.
In our code, the given number was 5. You can obviously adjust this number to your liking.
The result is 25 as the given number was 5, and this number can be changed as per the demand to get a different result.
So, in this article, we have learned How to square a number in JavaScript. I hope you might have found it useful.
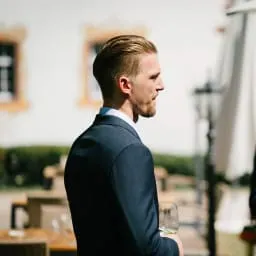
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!