In this post, we will see how to multiply two lists in Python. We want to find the product of list elements at corresponding positions.
Simply put, we have to perform the element-wise multiplication. So, let’s get started.
Multiply two Lists in Python by Using a Loop
One straightforward approach is to iterate over both lists, compute the product of values at the same index and append it to a new list. Let’s see an example.
list_one = [3, 4, 5 ,6, 8, 9] list_two = [4, 5, 1, 0, 6] product = [] for el1, el2 in zip(list_one, list_two): product.append(el1*el2) print("The product of two lists is: ", product)
Output
The product of two lists is: [12, 20, 5, 0, 48]
In the above example, we iterate over lists using the zip() method. It takes iterables (lists) and returns an iterator of tuples.
Moreover, make sure to run a loop n times, where n is the length of the smaller list.
The zip() method takes care of that as you can notice that list_one has six items and list_two has five, but the product list has five values only.
Multiply two Lists in Python by Using List Comprehension
We can also use the list comprehension to perform element-wise multiplication. This method should be preferred over the previous one because it is easier and concise. Let’s see.
list_one = [3, 4, 5 ,6, 8, 9] list_two = [4, 5, 1, 0, 6] product = [el1*el2 for el1, el2 in zip(list_one, list_two)] #element-wise multiplication print("The product of two lists is: ", product)
Output
The product of two lists is: [12, 20, 5, 0, 48]
See, this is a short and simple approach.
Multiply two Lists in Python by Using NumPy
Another method is to use the NumPy library. First, we convert both lists to NumPy arrays or ndarrays, i.e., arr1 and arr2.
Then, we multiply two arrays like we do numbers, i.e., arr1*arr2. Finally, we convert the ndarray to a list.
However, the length of both lists needs to be the same. Otherwise, we will get an error. Let’s see.
import numpy as np list_one = [3, 4, 5 ,6, 8, 9] list_two = [4, 5, 1, 0, 6,] n = min(len(list_one), len(list_two)) #get the minimum length #convert to numpy arrays arr1 = np.array(list_one) arr2 = np.array(list_two) product = arr1[:n]*arr2[:n] #element-wise multiplication #convert numpy array to list product = product.tolist() print("The product of two lists is: ", product)
Output
The product of two lists is: [12, 20, 5, 0, 48]
Here, we take the length of the smaller array and multiply only that number of elements.
NumPy is a powerful library for scientific computations. It provides several methods and tools to create and work with arrays efficiently.
Therefore, if we want to calculate the element-wise product of large data, then using NumPy will be very efficient.
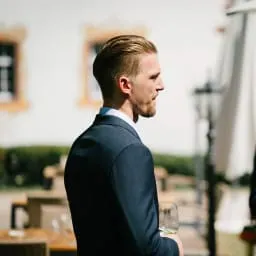
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!