In this post, we will learn how to multiply variables in Python. Usually, when we multiply two variables, we use x×y, where x and y are variables.
However, in most programming languages, including Python, we use the * (asterisk) sign to multiply variables instead of ×. So, to take the product of two variables, we use x*y. Simple, right?
Let’s have a look at an example:
x = 2 y = 4 result = x*y print("Result:", result)
Output
Result: 8
In the above example, x contains 2, and y holds 4. We take the product, i.e., 2*4=8, and store it in the result variable. Finally, we display it.
How to Multiply Variables in Python: Variables of type int or float
One thing to keep in mind while multiplying variables is that their types should be compatible.
So, if we want to perform arithmetic multiplication, all variables must be numbers, i.e., either integers or floating-point numbers.
Otherwise, the program will throw an error or provide unexpected results.
x = 2.5 y = 3 z = 7.5 result = x*y*z print("Result:", result)
Output
Result: 56.25
Here, x and z are floating-point numbers, and y is an integer. We get the correct result that is of type float.
How to Multiply Variables in Python: Variables of type int and string
Consider the following example.
x = 2 y = 'abc' result = x*y print("Result:", result)
Output
Result: abcabc
In the above example, x is of type integer and y of type string. In this case, using the * repeats the string by (x-1) times.
Therefore, we get the output abcabc, i.e., the string abc gets repeated one time.
x = 3 y = '4' result = x*y print("Result:", result)
Output
Result: 444
If you actually want to multiply numbers and don’t want the repetition, then convert the variable y to an integer using the int() method.
x = 3 y = '4' result = x*int(y) print("Result:", result)
Output
Result: 12
How to Multiply Variables in Python: Variables of type string or float
Multiplying a string by a string or a floating-point number will cause an error.
x = 3.2 y = '4' result = x*y print("Result:", result)
Output 1
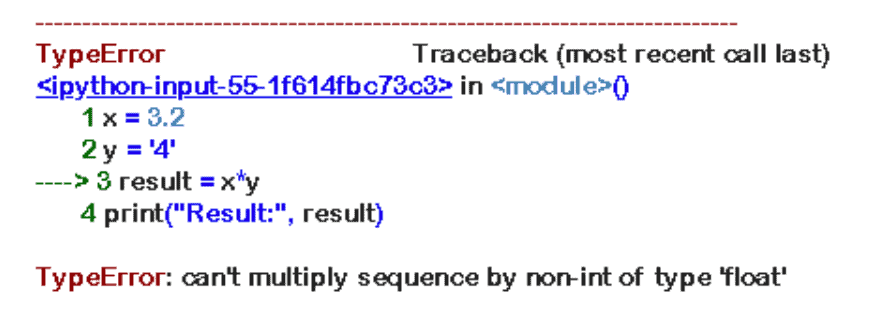
Output 1 Multiplying Variables in Python
x = '333' y = '4' result = x*y print("Result:", result)
Output 2
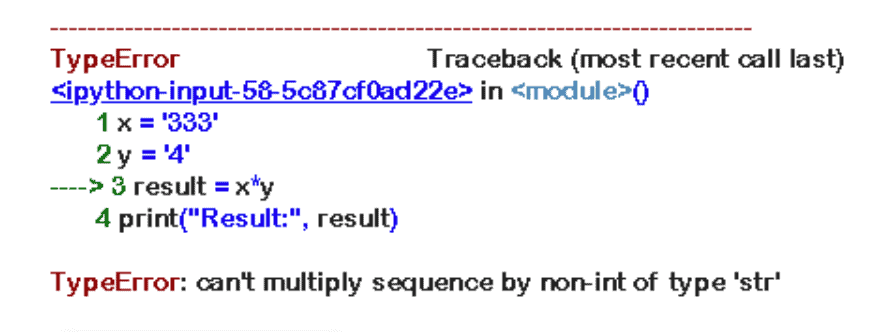
Output 2 Multiplying Variables in Python
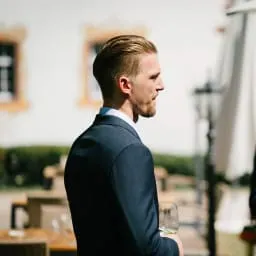
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!