%s is an argument specifier in Python and is used for string formatting. It borrows its syntax from the C language. Simply put,
it lets you add a value inside a string.
The value can be a string or any object that can get converted into a string, for example, a number, a list, etc.
All string values
Consider the following example.
name = input("Please insert your name: ") song = input("What is your favorite song? " ) print("Hello %s! Would you like to listen to %s?" %(name, song))
In the above example, we take the name and the favorite song from the user and display a message using these values.
Moreover, we put %s as a placeholder at those places where you want values of variables.
A tuple containing values follows the format string, i.e., %(name, song). Remember to insert values in the same order you want to display them. In this case, the name will come first, and then the song.
A sample output of the above example is given below.
Please insert your name: ashton What is your favorite song? Perfect Hello ashton! Would you like to listen to Perfect?
As you can see, this works as expected.
A single value
If we only have a single %s, then we can write a value without a tuple. Let’s see.
name = input("Please insert your name: ") print("Hello %s!" % name)
Output
Please insert your name: Agar Hello Agar!
Objects with a string representation
As already mentioned, a value can be any object that can be converted into a string. Let’s take an example.
name = "Smith" score = [70, 80, 90, 100] print("The score of %s in the last four matches: %s" % (name, score))
Output
The score of Smith in the last four matches: [70, 80, 90, 100]
As you can observe, we place a string and a list using the %s argument specifier. It converts the list to a string automatically.
Let’s take the same example and do it using the concatenation operator.
name = "Smith" score = [70, 80, 90, 100] print("The score of " + name + " in the last four matches " + str(score))
Output
The score of Smith in the last four matches [70, 80, 90, 100]
Here, unlike %s, we explicitly convert the list to a string and use + at every place we want to add a value.
TypeError
Moreover, the number of argument specifiers needs to be the same as the number of values in the tuple. If they are not, you will get an error. Let’s see.
name = input("Please insert your name: ") song = input("What is your favorite song? " ) print("Hello %s! Would you like to listen to %s?" %(name))
Output
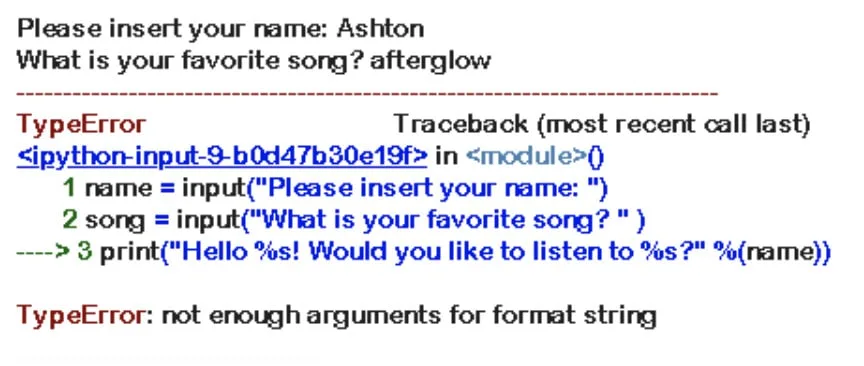
What does %s in Python mean
As you can see in the above output, the program throws a TypeError.
Mapping key
Instead of remembering the order in which you want to insert values, you can pass a mapping key to %s. Consider the following example to understand this concept.
name = "Ashton Agar" age = 20 print("My name is %(name)s and my age is %(age)s." %{"age":age, "name":name})
Output
My name is Ashton Agar and my age is 20.
In the above example, we pass a dictionary containing (key, value) pairs instead of a tuple. Moreover, the key is placed in between the % and s, which gets replaced by its value later. Therefore, we do not need to remember the order.
%s is an old method of formatting strings. Better techniques such as format() and f-strings have been introduced that are easier to use and provide more functionalities.
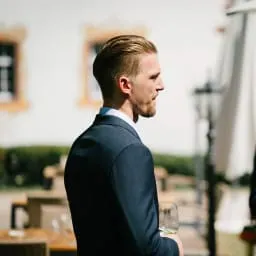
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!