In this article, we will see how to reverse a string in Python.
For example, if the given string is “cricket”, we want to get “tekcirc”. There are multiple ways to achieve that. Let’s see some of the methods.
1. Loop and Concatenation
One straightforward approach is to loop through the string from the end to the start and concatenate each character in a new variable (if you want to check if a variable already exists, have a look at this article: Check if a variable exists in Python). Have a look at the code below.
my_str = "cricket" reversed="" for i in range(len(my_str)-1, -1, -1): #iterate from end to start reversed += my_str[i] print("Reversed string:", reversed)
Output
Reversed string: tekcirc
2. Slicing
Another cool way is to use string slicing. Let’s see how.
my_str = "cricket" reversed = my_str[len(my_str)-1: : -1] print("Reversed string:", reversed)
Output
Reversed string: tekcirc
Slicing takes three parameters, start, end, and step. A negative step slices the list in the opposite direction. In the above code, we start from the last character of the string and go one step backward till we reach the end, i.e., index 0.
Consider the following equivalent representations of the above code.
my_str = "cricket" reversed = my_str[: : -1] print("Reversed string:", reversed) reversed = my_str[-1: : -1] print("Reversed string:", reversed)
Output
Reversed string: tekcirc Reversed string: tekcirc
3. join() and reversed()
We can reverse a string using Python’s join and reversed method. The reversed() method returns an iterator that contains the input sequence in reverse order.
The join() method takes an iterable and returns a string in which all items of an iterable are joined by a separator.
We can pass our string to the reserved() method. It will provide us an iterator that contains characters in reverse order. We will pass this as an argument to join() with an empty separator to give us a reversed string.

Reverse a string in Python join() and reversed()
my_str = "cricket" reversed = "".join(reversed(my_str)) print("Reversed string:", reversed)
Output
Reversed string: tekcirc
Conclusion
There are multiple ways to reverse a string in Python. In this article, we had a look at “Loop and Concatenation”, “Slicing” as well as join() and reversed().
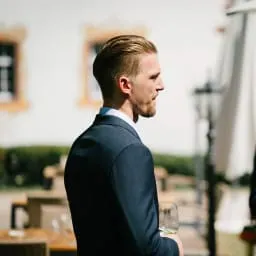
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!