In this post, we will see how to add space in Python. Specifically, we will cover the following topics:
- Add a space between variables in print()
- Add spaces to the left and right sides of a string
- Add spaces at the beginning of a string
- Add spaces at the end of a string
- Add spaces between characters in a string
- Add a space between string items
So, without further ado, let’s get started.
Add a space between variables in print()
Often, when we print variables, we want to separate them by a space to make them more readable. If variables in print() are separated by a comma, a space gets automatically added between them. Let’s take an example.
a = 4 b = 3 print(a, b) fname = "ashton" lname = "agar" print("His name is", fname, lname)
Output
4 3 His name is ashton agar
Next, we will see how to add spaces at different places in a string.
Add spaces to the left and right sides of a string
To add space to the left and right sides, we can use the center() method. It takes the length of the returned string. Moreover, it is invoked on a string and outputs a new one. Consider the following example.
name = "ashton" format = name.center(len(name)+8) print(format)
Output
ashton
In the above example, the new string’s length is 14, i.e., 8 for spaces and 6 for name length. 4 spaces get added to the left and 4 to the right.
Add spaces at the beginning of a string
We can use the rjust() method to add spaces at the beginning only. It right aligns the given string. It takes the length of the formatted string like the center() method does. Let’s see.
name = "ashton" format = name.rjust(len(name)+4) #add four spaces at the beginning print(format)
Output
ashton
Add spaces at the end of a string
The ljust() method, on the other hand, can be used to add spaces to the end. Let’s see.
name = "ashton" format = name.ljust(len(name)+4) #add four spaces to the end print(format)
Output
ashton
Add spaces between characters in a string
Let’s see how to separate each character in a string by a space. For that, we can use the join() method.
The join() method takes an iterable (list, string) and returns a string in which all items of an iterable are joined by a separator.
Here, we will pass our input string to join() and use space as a separator. Let’s see.
string = "cricket" formatted = " ".join(string) #separate each character by a space print(formatted)
Output
c r i c k e t
As you can see, we add space between each character of the string.
Add a space between string items
Using the join() method, we can also add space between two or more string items. Let’s see.
str1 = "cricket" str2 = "football" str3 = "hockey" string = " ".join([str1, str2, str3]) print(string)
Output
cricket football hockey
In the above example, we pass a list containing string items to the join() method. It returns a new string having the list items joined by a space.
If you only want to display (print) strings in such a way, you can use the print() method and separate them using a comma.
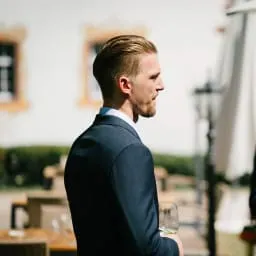
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!