In this post, we will see how to access the last element in a Python list.
Python provides us with simple and efficient ways to do that. Let’s talk about them one by one.
1.Using the len() method to retrieve the Last Element of a Python list
We can find the total number of elements in a list using the len() method. We know that the index starts from 0.
If a list has length 3, then the first element will be at index 0, the second item at index 1, and the third (last) one at index 2.
If we generalize this, if n is the list length, then the last item will be at index n-1. Let’s take an example.
lst = [2, 5 , 6, 3, 8, 9] n = len(lst) #get the length last_el = lst[n-1] #get the last element print("The last element of the list is:", last_el)
Output
The last element of the list is: 9
2. Using a negative index to get the last element of a list in Python
Python allows us to access list items from the end as well directly. We can do so using a negative index. So, the last element exists at index -1, the second last item at index -2, and so on. Consider the following example.
lst = [2, 5 , 6, 3, 8, 9] last_el = lst[-1] #get the last element print("The last element of the list is:", last_el)
Output
The last element of the list is: 9
3. Using the pop() method to fetch the last item in a Python list
We can also retrieve the last element using the pop() method.
However, it not only returns the last item but deletes it from the list, too. Therefore, you have to be careful before using this method.
lst = [2, 5 , 6, 3, 8, 9] last_el = lst.pop() print("The last element of the list is:", last_el) print("The updated list:", lst)
Output
The last element of the list is: 9 The updated list: [2, 5, 6, 3, 8]
As you can observe in the above example, the last item gets deleted.
4. Using the reversed() method and the next() method to catch the last item in a Python list
Another method to get the last element of a Python list is to reverse the list and then access the first element.
We can reverse the list using the reversed() method.
It returns an iterator that contains the input sequence in reverse order. Since it returns an iterator, we convert it to a list using the list() method and then get the first element.
lst = [2, 5 , 6, 3, 8, 9] rev_iter = reversed(lst) #reverse the list rev_list = list(rev_iter) #converts an iterator to list print("The last element of the list is:", rev_list[0])
Output
The last element of the list is: 9
Instead of converting to a list, we can use the next() method to get the next item from an iterator.
lst = [2, 5 , 6, 3, 8, 9] rev_iter = reversed(lst) #reverse the list last_el = next(rev_iter) print("The last element of the list is:", last_el)
Output
The last element of the list is: 9
As you can observe, this is not the most efficient approach.
Happy coding!
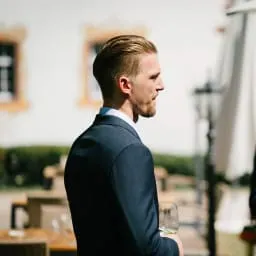
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!