An asterisk (*) operator in Python is quite powerful and can do many things. In this post, we will uncover its powers. So, without further ado, let’s get started.
Multiplication and Power
The * operator can multiply two numbers. If we use double asterisks (**) between operands, then it will perform the exponential (power) calculation. For example, 3**2 = 9, i.e., it calculates 3 to the power 2. Let’s see their examples.
x = 4 y = 5 product = x*y #* as a multiplication operator power = x**3 #* to calculate power print(product, power)
Output
20 64
Repetition
When the * operator is used between an iterable (list, string, etc.) and an integer x, it repeats that sequence by x-1 times. Consider the following example.
x = 4 lst = [2]*x #repeats 2 in the list x-1 times print(lst) string = "abc"*x #repeats abc x-1 times print(string)
Output
[2, 2, 2, 2] abcabcabcabc
Unpacking iterables and dictionaries
The unpacking operator * can unpack an iterable. For dictionaries, use **. Let’s see.
list1 = ["football", "basketball", "cricket"] list2 = ["hockey", "volleyball"] sports = [*list1, *list2] #unpacking list1 and list2 to merge them into a new list print(sports)
Output
['football', 'basketball', 'cricket', 'hockey', 'volleyball']
Consider another example.
list1 = ["football", "basketball", "cricket"] first, *others = list1 print("The first item:", first) print("Rest of the items:", others)
Output
The first item: football Rest of the items: ['basketball', 'cricket']
Let’s see how to unpack dictionaries.
#unpacking dictionaries dictt1 = {"name":"Ashton", "age": 25} dictt2 = {"cpga": 4.5} dictt = {**dictt1, **dictt2} print(dictt)
Output
{'name': 'Ashton', 'age': 25, 'cpga': 4.5}
Unpacking iterables into a function call
If we want to pass items of an iterable (lists, string) separately as arguments to a function, you can do so using the * operator. Consider the following example.
name = "ashton agar" print("The letters in the string are:", *name)
Output
The letters in the string are: a s h t o n a g a r
Varying number of positional arguments
The * operator allows you to pass any number of positional arguments to a function. Moreover, these arguments get passed as a tuple. Let’s see.
def calculateAverage(*values): n = len(values) summ =0 for i in range(0, n): summ += values[i] return summ/n avg = calculateAverage(2,6, 7, 8, 8) print("The average is:", avg)
Output
The average is: 6.2
In the above example, we create a function to calculate the average of numbers passed to it. You can observe here that we are not limiting the arguments passed to it. We do that using the asterisk operator.
Varying number of keyword arguments
While the * operator allows us to pass any number of positional arguments, the ** operator can be used to pass a variable number of keyword arguments. Let’s see.
def test(**info): print(info) test(name="Ashton", age=3)
Output
{'name': 'Ashton', 'age': 3}
Keyword-only parameter
If you want to have a keyword-only parameter in your function, you can use * to do that. Consider the following example to see how.
def test(name, age, *, grade): print(name, age, grade) test("ashton", 18, grade=9)
Output
ashton 18 9
In the above example, the test() function takes three arguments. Variables name and age are positional, but grade is a keyword-only argument.
def test(name, age, *, grade): print(name, age, grade) test("ashton", 18, 9)
Output
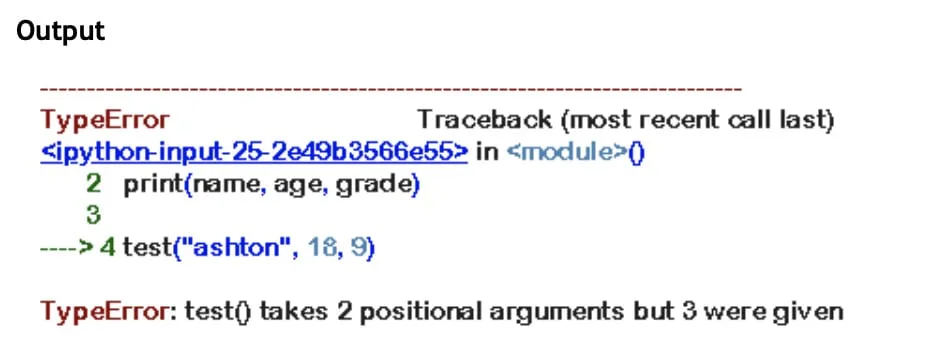
What does * do in Python?
As you can see, you get an error when you don’t pass the keyword argument properly.
We have seen that the * operator has many meanings. So, I would encourage you guys to play around with it to understand it better.
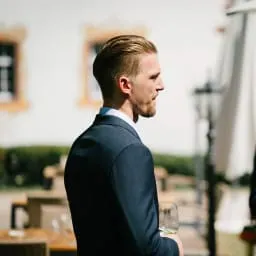
Hey guys! It’s me, Marcel, aka Maschi. On MaschiTuts, it’s all about tutorials! No matter the topic of the article, the goal always remains the same: Providing you guys with the most in-depth and helpful tutorials!